介绍
栈
栈是一种特殊的线性表。其特殊性在于限定插入和删除数据元素的操作只能在线性表的一端进行。如下所示:
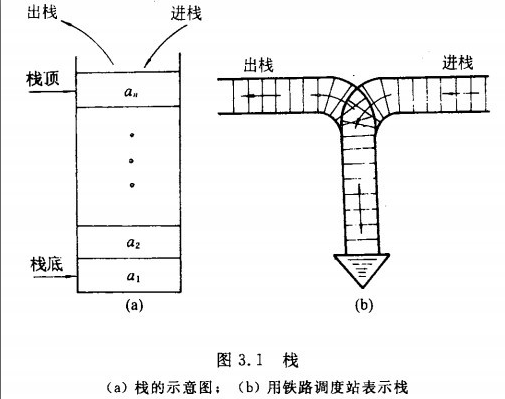
结论:先进后出 && 后进先出(Last In First Out),简称为LIFO线性表。
例子:手机任务的返回栈、食堂餐盘从下到上叠起来,送给洗盘子的人,从上到下洗
队列
队列(Queue)也是一种运算受限的线性表,它的运算限制与栈不同,是两头都有限制,插入只能在表的一端进行(只进不出),而删除只能在表的另一端进行(只出不进),允许删除的一端称为队尾(rear),允许插入的一端称为队头 (Front),如图所示:

结论:队列的操作原则是先进先出的,所以队列又称作FIFO表(First In First Out)
例子:各种排队
纯 C 用法(函数)
栈
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| #include <stdio.h>
#define MAX_SIZE 1000
int _data[MAX_SIZE], _size = 0;
bool empty() { return _size != 0; }
void push(int x) { if (_size < MAX_SIZE-1) { _data[_size++] = x; } }
void pop() { if (_size) { _data[--_size] = 0; } }
int size() { return _size; }
int front() { return _size ? _data[0] : 0; }
int back() { return _size ? _data[_size-1] : 0; }
int main() {
push(100); printf("%d\n", back()); push(20); printf("%d\n", back()); }
|
数组转指针:
_data\[([^M].*)\]
替换*(_data + \1)
纯C用法(结构体)
栈
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| #include <stdio.h>
struct Stack { int _data[1000]; int _size;
Stack() { _size = 0; }
Stack(Stack& q) { _size = q.size(); for (int i = 0; i < _size; i++) _data[i] = q.at(i); }
bool empty() { return !_size; }
int size() { return _size; }
int at(int x) { return (x>=0 && x < _size) ? _data[x] : 0; }
void push(int x) { if (_size < 1000) _data[_size++] = x; }
void pop() { if (_size) { for (int i = 0; i < _size-1; i++) _data[i] = _data[i+1]; --_size; } }
int front() { return _size?_data[0] : 0; }
int back() { return _size?_data[_size-1] : 0; }
void clear() { _size = 0; } };
int main() { Stack s;
s.push(100); printf("%d\n", s.back()); s.push(20); printf("%d\n", s.back());
Stack s2(s); printf("%d\n", s.back()); s2.clear(); }
|
C++ 用法
头文件
1 2
| #include <queue> #include <stack>
|
定义方式
1 2
| queue<int> q; statck<int> q;
|
常用操作
栈
1 2 3 4 5
| s.empty() s.size() s.pop() s.top() s.push(x)
|
队列
1 2 3 4 5 6
| q.empty() q.size() q.pop() q.front() q.push(x) q.back()
|
智能指针
迭代器 iterator
1 2 3
| stack<int>::iterator i; for (i = s.begin(); i != s.end(); ++i) cout << *i << endl;
|
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| #include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <queue> #include <stack> #include <vector> using namespace std; int main() { queue<int> q; stack<char> s; char a = 'a', b = 'b'; q.push(1); cout << "push 1" << endl; cout << "empty:" << q.empty() << endl; cout << "push 2" << endl; q.push(2); cout << "front:" << q.front() << endl; q.pop(); cout << "pop" << endl; cout << "front:" << q.front() << endl; q.pop(); cout << "pop" << endl; cout << "empty:" << q.empty() <<endl;
cout << "-----" << endl; s.push(a); cout << "push " << a << endl; cout << "size:" << s.size() << endl; cout << "top:" << s.top() << endl; s.push(b); cout << "push " << b << endl; cout << "top:" << s.top() << endl; s.pop(); cout << "pop" << endl; cout << "top:" << s.top() << endl; }
|